Simple texture in OpenGL
WASM demo
Note. If you draw a PNG texture you should use GL_RGBA
in the glTexImage2D
function but if you draw a JPG texture you should use GL_RGB
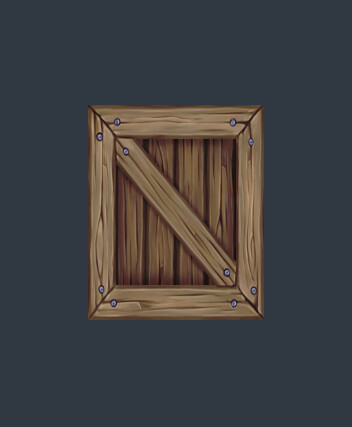
main.cpp
#include <SDL3/SDL.h>
#include <SDL3/SDL_main.h>
#include <SDL3/SDL_opengles2.h>
#include <iostream>
#ifdef __EMSCRIPTEN__
#include <emscripten.h>
#include <emscripten/html5.h>
#endif // __EMSCRIPTEN__
#define STB_IMAGE_IMPLEMENTATION
#include "stb_image.h"
struct AppContext
{
SDL_Window *window;
SDL_GLContext glcontext;
SDL_bool app_quit = SDL_FALSE;
};
const char *vertexShaderSource =
"attribute vec2 aPosition;\n"
"attribute vec2 aTexCoord;\n"
"varying vec2 vTexCoord;\n"
"void main()\n"
"{\n"
" gl_Position = vec4(aPosition, 0.0, 1.0);\n"
" vTexCoord = aTexCoord;\n"
"}\n";
const char *fragmentShaderSource =
"#ifdef GL_ES\n"
"precision mediump float;\n"
"#endif\n"
"uniform sampler2D uSampler;\n"
"varying vec2 vTexCoord;\n"
"void main()\n"
"{\n"
" gl_FragColor = texture2D(uSampler, vTexCoord);\n"
"}\n";
// Helper function for creating shaders
GLuint createShader(const char *shaderSource, int shaderType)
{
GLuint shader = glCreateShader(shaderType);
glShaderSource(shader, 1, &shaderSource, NULL);
glCompileShader(shader);
GLint status;
glGetShaderiv(shader, GL_COMPILE_STATUS, &status);
if (status == GL_FALSE)
{
GLint maxLength = 0;
glGetShaderiv(shader, GL_INFO_LOG_LENGTH, &maxLength);
std::vector<GLchar> errorLog(maxLength);
glGetShaderInfoLog(shader, maxLength, &maxLength, &errorLog[0]);
glDeleteShader(shader); // Don't leak the shader
std::cout << &(errorLog[0]) << std::endl;
std::cout << shaderSource << std::endl;
}
return shader;
}
// Helper function for creating a shader program
GLuint createShaderProgram()
{
GLuint program = glCreateProgram();
GLuint vShader = createShader(vertexShaderSource, GL_VERTEX_SHADER);
GLuint fShader = createShader(fragmentShaderSource, GL_FRAGMENT_SHADER);
glAttachShader(program, vShader);
glAttachShader(program, fShader);
glLinkProgram(program);
glUseProgram(program);
return program;
}
// Load a triangle to the video card
void initVertexBuffers(GLuint program)
{
float vertPositions[] = {
// First triangle
-0.5f, -0.5f,
0.5f, -0.5f,
-0.5f, 0.5f,
// Second triangle
-0.5f, 0.5f,
0.5f, -0.5f,
0.5f, 0.5f
};
GLuint vertPosBuffer;
glGenBuffers(1, &vertPosBuffer);
glBindBuffer(GL_ARRAY_BUFFER, vertPosBuffer);
int amount = sizeof(vertPositions) / sizeof(vertPositions[0]);
glBufferData(GL_ARRAY_BUFFER, amount * sizeof(GLfloat),
vertPositions, GL_STATIC_DRAW);
GLint aPositionLocation = glGetAttribLocation(program, "aPosition");
glVertexAttribPointer(aPositionLocation, 2, GL_FLOAT, GL_FALSE, 0, 0);
glEnableVertexAttribArray(aPositionLocation);
float texCoords[] = {
// First triangle
0.f, 1.f,
1.f, 1.f,
0.f, 0.f,
// Second triangle
0.f, 0.f,
1.f, 1.f,
1.f, 0.f
};
GLuint texCoordBuffer;
glGenBuffers(1, &texCoordBuffer);
glBindBuffer(GL_ARRAY_BUFFER, texCoordBuffer);
amount = sizeof(texCoords) / sizeof(texCoords[0]);
glBufferData(GL_ARRAY_BUFFER, amount * sizeof(GLfloat),
texCoords, GL_STATIC_DRAW);
GLint aTexCoordLocation = glGetAttribLocation(program, "aTexCoord");
glVertexAttribPointer(aTexCoordLocation, 2, GL_FLOAT, GL_FALSE, 0, 0);
glEnableVertexAttribArray(aTexCoordLocation);
}
GLuint createTexture(const char *path)
{
int h_image, w_image, cnt;
unsigned char *data = stbi_load(path, &w_image, &h_image, &cnt, 0);
if (data == NULL)
{
std::cout << "Failed to load the image: " << path << std::endl;
return 0;
}
glEnable(GL_TEXTURE_2D);
GLuint texture;
glGenTextures(1, &texture);
glBindTexture(GL_TEXTURE_2D, texture);
{
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP_TO_EDGE);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP_TO_EDGE);
// GL_NEAREST - for pixel graphics
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR);
glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA, w_image, h_image, 0, GL_RGBA, GL_UNSIGNED_BYTE, data);
}
stbi_image_free(data);
return texture;
}
int SDL_Fail()
{
SDL_LogError(SDL_LOG_CATEGORY_CUSTOM, "Error %s", SDL_GetError());
return -1;
}
int SDL_AppInit(void **appstate, int argc, char *argv[])
{
// init the library, here we make a window so we only need the Video capabilities.
if (SDL_Init(SDL_INIT_VIDEO))
{
return SDL_Fail();
}
// create a window
SDL_Window *window = SDL_CreateWindow("Window", 352, 430,
SDL_WINDOW_OPENGL | SDL_WINDOW_RESIZABLE);
if (!window)
{
return SDL_Fail();
}
SDL_GLContext glcontext = SDL_GL_CreateContext(window);
if (!glcontext)
{
return SDL_Fail();
}
// Create a shader program and load a triangle to the video card
GLuint program = createShaderProgram();
initVertexBuffers(program);
const char *texturePath = "./box.png";
GLuint texture = createTexture(texturePath);
// print some information about the window
SDL_ShowWindow(window);
{
int width, height, bbwidth, bbheight;
SDL_GetWindowSize(window, &width, &height);
SDL_GetWindowSizeInPixels(window, &bbwidth, &bbheight);
SDL_Log("Window size: %ix%i", width, height);
SDL_Log("Backbuffer size: %ix%i", bbwidth, bbheight);
if (width != bbwidth)
{
SDL_Log("This is a highdpi environment.");
}
}
// set up the application data
*appstate = new AppContext {
window,
glcontext,
};
SDL_Log("Application started successfully!");
return 0;
}
int SDL_AppEvent(void *appstate, const SDL_Event *event)
{
auto *app = (AppContext *)appstate;
switch (event->type)
{
case SDL_EVENT_QUIT:
{
app->app_quit = SDL_TRUE;
break;
}
default:
{
break;
}
}
return 0;
}
int SDL_AppIterate(void *appstate)
{
auto *app = (AppContext *)appstate;
glClearColor(0.188f, 0.22f, 0.255f, 1.f);
glClear(GL_COLOR_BUFFER_BIT);
glDrawArrays(GL_TRIANGLES, 0, 6);
SDL_GL_SwapWindow(app->window);
return app->app_quit;
}
void SDL_AppQuit(void *appstate)
{
auto *app = (AppContext *)appstate;
if (app)
{
SDL_GL_DeleteContext(app->glcontext);
SDL_DestroyWindow(app->window);
delete app;
}
SDL_Quit();
SDL_Log("Application quit successfully!");
}