jrxx
1
// Hi hello, I need to click on rotate texture instead of rectangle R what do I do?
SDL_Point p = { 100,100};
SDL_Rect r = { 100,100,60,100 };
sp.x = rw.x;
sp.y = rw.y;
SDL_RenderCopyEx(renderer, texture, NULL, &r, 50, &p, SDL_FLIP_NONE);
if (SDL_HasIntersection(&r, &mouse_rect)) {
SDL_RenderCopyEx(renderer, texture2, NULL, &r, 50, &p, SDL_FLIP_NONE);
}
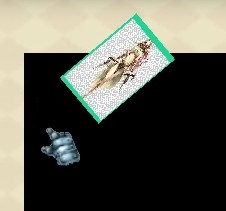
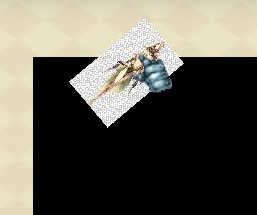
This is the code I use for detecting if a point lies inside a rotated rectangle:
+(BOOL)isPoint:(struct UXPOINT)pt // ptA, ptB and ptD define a rectangle
WithinA:(struct UXPOINT)ptA
WithinB:(struct UXPOINT)ptB
WithinD:(struct UXPOINT)ptD
{
float bax, bay, dax, day;
bax = ptB.x - ptA.x;
bay = ptB.y - ptA.y;
dax = ptD.x - ptA.x;
day = ptD.y - ptA.y;
if ((pt.x - ptA.x) * bax + (pt.y - ptA.y) * bay < 0.0) return FALSE;
if ((pt.x - ptB.x) * bax + (pt.y - ptB.y) * bay > 0.0) return FALSE;
if ((pt.x - ptA.x) * dax + (pt.y - ptA.y) * day < 0.0) return FALSE;
if ((pt.x - ptD.x) * dax + (pt.y - ptD.y) * day > 0.0) return FALSE;
return TRUE;
}
It’s in Objective C but it’s easy to convert to C. My UXPOINT is just a struct of an x and y float